Example 10 Using Sensors
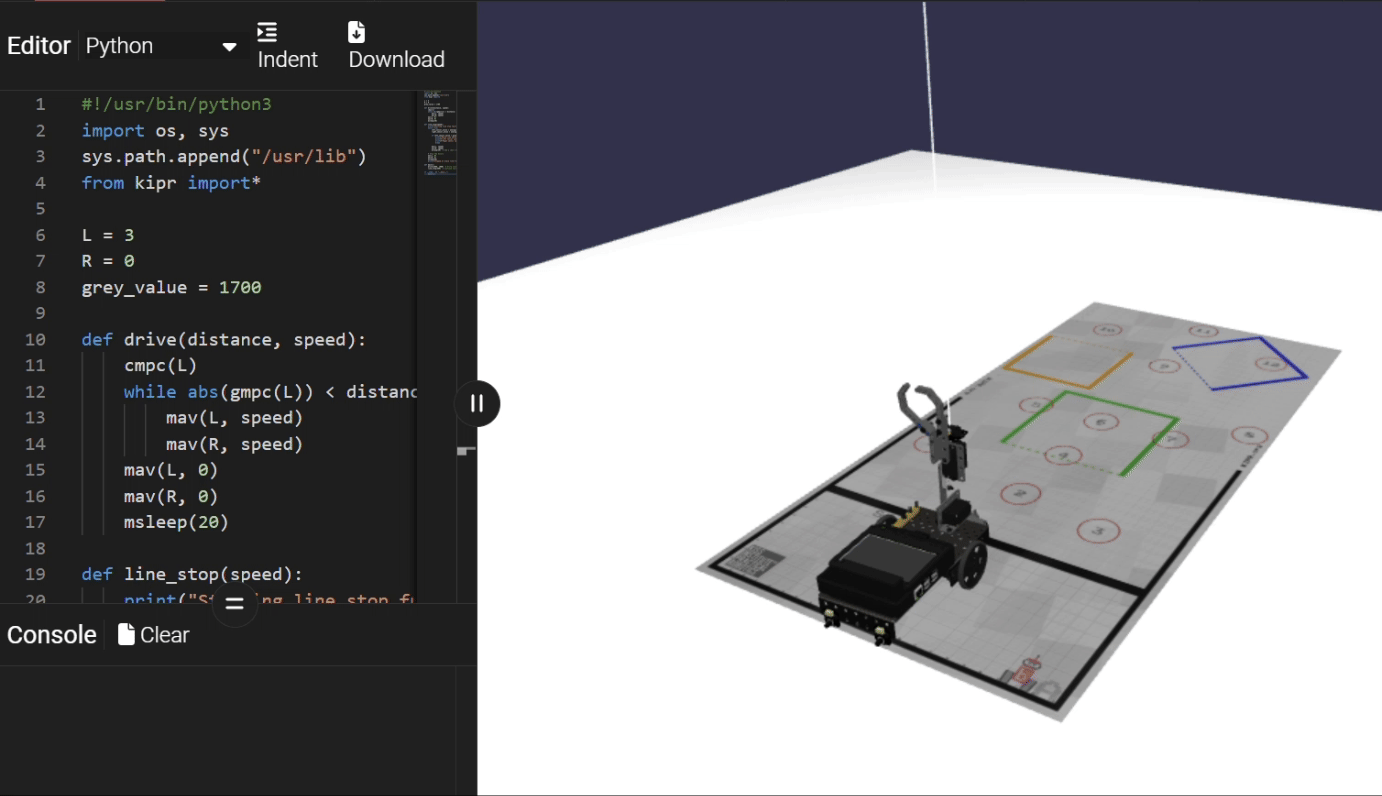
What does this code do?
In this code the robot moves forward until it detects a black line.
Functions Used
cmpc( clear_motor_position_counter )
abs( absolute )
gmpc( get_motor_position_counter )
Function Implementation
1#!/usr/bin/python3
2import os, sys
3sys.path.append("/usr/lib")
4from kipr import*
5
6L = 3
7R = 0
8grey_value = 1700
9
10def drive(distance, speed):
11 cmpc(L)
12 while abs(gmpc(L)) < distance:
13 mav(L, speed)
14 mav(R, speed)
15 mav(L, 0)
16 mav(R, 0)
17 msleep(20)
18
19def line_stop(speed):
20 print("Starting line stop function:")
21 while True:
22 left_sensor_value = analog(L)
23 right_sensor_value = analog(R)
24
25 if left_sensor_value < grey_value or right_sensor_value < grey_value:
26 print("Black line detected!")
27 print(f"Left sensor (port {L}) value: {left_sensor_value}")
28 print(f"Right sensor (port {R}) value: {right_sensor_value}")
29 break
30
31 mav(L, speed)
32 mav(R, speed)
33 msleep(20) # Add a small delay to prevent too frequent updates
34
35 # Stop the motors
36 mav(L, 0)
37 mav(R, 0)
38 msleep(50)
39 print("Stopped at black line!")
40
41def main():
42 drive(2000, 1000) # Drive forward for 2000 ticks with speed 1000
43 line_stop(500) # Continue moving forward until black line is detected
44
45if __name__ == "__main__":
46 main()